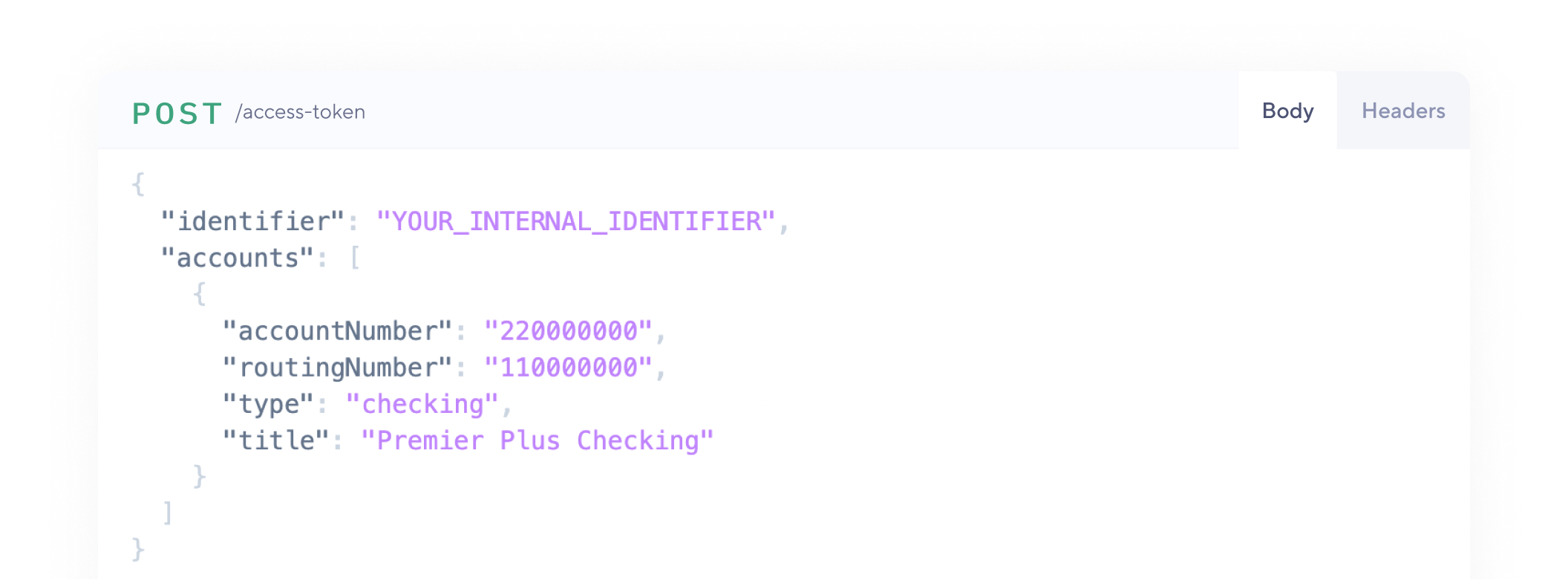
PayLink API Reference
Atomic's API is built around RESTful principles, using JSON encoded request and response bodies.
To get going quickly, we recommend using Postman - an API collaboration tool. Atomic provides a public workspace which you can fork into your own workspace. Use the button below to get started.
The workspace comes fully documented with:
- several useful flows using our APIs
- requests for all of the Atomic endpoints
- an environment containing placeholders for all the variables needed to connect to your Sandbox instance of Console
Authentication
Atomic uses a combination of API keys and access tokens to authenticate requests. Your API keys carry many privileges, so be sure to keep them secure! Do not share your secret API keys in publicly accessible areas such as GitHub, client-side code, etc.
API Key and Secret
You can retrieve and manage your API key and secrets on the credentials page on the Atomic Console. Include these headers in requests that are secured with your API Key and Secret:
Header | Description |
---|---|
x-api-key | API Key for your Atomic account |
x-api-secret | API Secret for your Atomic account |
Access Token
A publicToken
will be included in the headers of requests which are made via the end user from client-side code, e.g. searching for their employer or initializing a Task. The publicToken
defaults to a 24 hour expiry, but can be set to a minimum of 30 minutes or a maximum of 30 days by passing in the desired duration using the optional tokenLifetime
property in the call to /access-token
. The minimum is to allow for users to complete variations of the flows, such as MFA, which can take time.
Header | Description |
---|---|
x-public-token | Public token generated during access token creation. |
Errors
Our API returns standard HTTP success or error status codes. For errors, we will also include extra information about what went wrong encoded in the response as JSON. The various HTTP status codes we might return are listed below.
Code | Title | Description |
---|---|---|
200 | OK | The request was successful. |
400 | Bad Request | The request was unacceptable. |
401 | Unauthorized | Missing or invalid credentials. |
404 | Not Found | The resource does not exist. |
422 | Validation Error | A validation error occurred. |
429 | Too Many Requests | Too may requests, please try again later. |
50X | Internal Server Error | An error occurred with our API. |
Access Token
An AccessToken
grants access to Atomic's API resources for an end user when its value is used as the x-public-token
header.
To create an access token pass in an identifier and identity data for the end user as well as associated account or card details.
identifier
for the user is required when creating an AccessToken
to initialize the Transact SDK. - If you control all the account and/or card data you would like the end user to select from when updating their payments in the vendor systems, use the Create an Access Token.
- If your card data is managed by a 3rd party, we have a flow where we can pull the tokenized card data. Replace the card details object in the Create an Access Token with the data as shown in the Access Token with BaaS passthrough section.
- If you want to transmit card data after the user has authenticated with the merchant, use the Create an Access Token API, but
lastFour
andtitle
are the only required properties on the card object. - To transmit bank account data after the user has authenticated with the merchant, use the Create an Access Token api, but provide
accountNumberLastFour
in place ofaccountNumber
.
Create Access Token
This API is how a flow through the Atomic experience is initialized. You will first create an AccessToken
which is used to instantiate the Transact SDK. There are variations on how this AccessToken
is created and will vary depending on the operation to be called.
For PayLink Manage use cases, you only need to pass an identifier
for the user.
For PayLink Switch use cases, you will need to pass all of the data fields listed below.
pci.atomicfi.com
in production, and sandbox-pci.atomicfi.com
in sandbox. Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
Required Properties
identifier
string- A unique identifier from your system that will be used to reference this user. This could be a primary key used in your system or another unique identifier. Creating multiple access tokens with the same identifier will tie those tokens to the same user in Atomic's system.
cards
[CardDetails]- An array of card detail objects which the end user can select from to update their card on file. Details for either an account or a card must be provided to complete the operation.
accounts
[AccountDetails]- An array of account detail objects which the end user can select from to update their account on file. Details for one of either an ACH account or card must be provided to complete the operation.
identity
object- Details about the end user's identity. These details are regularly required to make an update to a vendor system.
Child Properties
Required Properties
firstName
string- End user's first name
lastName
string- End user's last name
postalCode
string- End user's postal code from their address
address
string- End user's street address
city
string- End user's home city
state
string- End user's home state. 2 digit state code is expected.
phone
string- End user's 10 digit phone number without
()
or-
PayLink Manage
POST
/access-tokenPayLink Switch
POST
/access-tokenBaaS passthrough variation
This version of the API is intended to be used when the calling application is not PCI compliant itself, but rather relies on a BaaS provider to handle the card data. See our guide on transmitting card data for details on whether or not this endpoint is for you.
Properties not explicitly defined in this section will function and be required in the same manner as the Create Access Token flow.
Required Properties
cards
[CardDetails]- An array of card detail objects which the end user can select from to update their card on file. Details for either an account or a card must be provided to complete the operation.
Child Properties
Required Properties
lastFour
string- The last four digits of a card number.
title
string- Title for the card
paymentService
object- Object containing details required for Atomic to connect to and utilitize a BaaS card provider
Child Properties
Required Properties
galileo
object- String which indicates the associated BaaS you use as your card provider. This example uses Galileo, if another other BaaS provider is required, please reach out to your contacts at Atomic.
Child Properties
Required Properties
apiLogin
string- Web service username, as provided by Galileo.
apiTransKey
string- Web service password, as provided by Galileo.
providerId
string- Galileo-issued provider identifier.
transactionId
string- A unique provider-generated ID to identify this API call. A UUID is preferred.
accountNo
string- The PRN, PAN or CAD of the account. For card-specific endpoints such as this one, the CAD is preferred. Do not use the PRN if more than one card has ever been associated with this account.
apiUrl
string- The production url that your organization uses to connect to Galileo.
PayLink Switch - BaaS passthrough
POST
/access-tokenResponse
Successfully creating an Access Token will return a payload with a data object containing a publicToken
. The publicToken
may be used to initialize the Transact SDK and is valid for tokenLifetime
seconds.
{
"data": {
"publicToken": "\"PUBLIC_TOKEN\""
}
}
Revoke Access Token
This endpoint is used to revoke an existing AccessToken
. This action invalidates the token, preventing it from being used to access Atomic's API resources.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
PUT
/access-token/:publicToken/revokeCompany
PayLink enables connectivity to merchant accounts, streaming services, and recurring bills to update payment methods and manage subscriptions. In this context, a 'Company' refers to the service or merchant that a user connects to in order to manage and update payment details.
Company Search
Use this endpoint to build a typeahead experience, as employed in our Transact SDK, or to get the company's _id
for deeplinking to the login section of Transact.
Search for a Company using a text query
. Searches can also be filtered by passing in additional properties detailed below.
Authentication can be handled via either the API Key and Secret method or the Access Token method.
POST
/company/searchAccounts
An Account
in the Atomic context refers to a connection authenticated by an end user to a third-party system. This connection enables both read and write operations on the linked system as authorized by the user. For instance, after linking a Netflix account, Atomic can retrieve account data or perform actions such as pausing the subscription or managing the user’s plan.
Get Accounts
This endpoint returns all Accounts associated with an end user.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
Optional Properties
supported
boolean
connected
boolean
GET
/pay-link/accountsResponse
The response includes an Account
object.
accounts
[Account]- An array of
Account
objects.Child Properties
Required Properties
_id
string- Unique identifier for the PayLink Account connected by the end user.
company
Company- An object containing details of the company to which the PayLink Account is connected.
Child Properties
Optional Properties
_id
stringUnique identifier for the company.name
stringThe name of the company.branding
objectThe branding information of the company. Useful if you are surfacing logos in your experience.color
stringThe color of the company. Atomic uses this color to style the experience - buttons and other UI elements. status
enum- The status of the account, either
initial
,connected
, ordisconnected
. If the account isinitial
, then the account has been selected but the user has not attempted to connect to it. If the account isconnected
then the account can be accessed. If the account isdisconnected
, then we cannot access the account with the given credentials. processing
boolean- A boolean value indicating whether or not the account is processing.
name
string- A name that can be used to display the account to the user. This field will be selected based of off the availablilty of data. In most cases it will either be
account.name
orcompany.name
. In cases where we cannot identify an appropriate name, it will be the stringUnknown Expense
. type
enum- An enum of the status of the account. Options include:
linked
,suggested
andunsupported
. Iflinked
, then the user has logged into the account and the user's data has been retrieved. Ifsuggested
, then the account has either been selected by the user or by our automated detection engine, but the user has not logged in yet. Ifunsupported
, then the account has been detected by our automation engine, but Atomic does not currently support integration for the account. account
Account- An object containing specific details of the account connected by the end user.
Child Properties
Required Properties
sourceId
string- An ID of the account pulled from the connected system.
type
enum- An enum of the type of account. Options include:
subscription
loan
policy
telecom
Optional Properties
name
stringThe name of the account.identities
[Identity]The identities connected to the account.Child Properties
Optional Properties
firstName
stringThe first name of the identity.lastName
stringThe last name of the identity.email
stringThe email associated with the identity.phone
stringThe phone number associated with the identity.address
stringThe address associated with the identity.address2
stringThe line 2 of the address associated with the identity.city
stringThe city of the address associated with the identity.state
stringThe state of the address associated with the identity.postalCode
stringThe postalCode of the address associated with the identity.billing
objectAn object containing the billing details of the account.Child Properties
Optional Properties
accountNumber
stringThe account number associated with the billing details for this account.amount
numberThe amount of the bill.dueDate
stringThe upcoming due date for the bill.autopay
objectitems
[BillingItems]history
[BillingHistory]paymentMethods
[PaymentMethod]An array of objects containing the payment methods associated with the account.Child Properties
Optional Properties
type
enumThe type of the payment method. One ofbank
,card
orpaypal
.lastFour
stringThe last four digits of the payment method.brand
stringThe brand of the payment method.isPrimary
booleanWhether the payment method is the primary payment method.description
stringThe description of the payment method.expiry
dateThe date the payment method expires.accountNumber
stringThe account number of the payment method. Only applicable fortype
ofbank
.routingNumber
stringThe routing number of the payment method. Only applicable fortype
ofbank
.data
objectThis object is dynamic based on the accounttype
.Child Properties
Optional Properties
plans
[Plan]The plans associated with the account. This field will be included if the accounttype
is eithersubscription
ortelecom
.Child Properties
Optional Properties
sourceId
stringThe source ID of the plan.status
stringThe status of the plan.title
stringThe title of the plan.description
stringThe description of the plan.freeTrial
booleanWhether the plan is on a free trial.usage
[Usage]The usage of the account. This field will be included if the accounttype
is eithersubscription
ortelecom
.Child Properties
Optional Properties
title
stringThe title of the usage.description
stringThe description of the usage.used
numberThe quantity of the plan used.limit
numberThe limit of the plan available to use.unit
stringThe units of usage of the plan. One ofgigabytes
,megabytes
,minutes
orkilowatts-per-hour
.lastUsed
dateThe last date the the plan was used. actions
[Actions]- The actions that can be performed on the account.
Child Properties
Optional Properties
id
stringThe ID of the action.type
enumAn enum representing the possible actions that can be performed on a PayLink Account. This comprehensive list includes all actions available for a company. However, each user will only see a subset of these actions, tailored to their specific account and plan.change-plan
cancel-plan
pause-plan
view-account
refresh
automated
booleanWhether or not the action is automated. Iftrue
, a task will be created to track and peform the automation. The status of the task can be monitorieed using webhooks. Iffalse
, then the user will perform the action on their device.
Optional Properties
suggestions
[Suggestions]An array of suggestion objects containing information about suggestions Atomic recommends presenting to the user based on the state of their account in the connected systemChild Properties
Required Properties
actions
[Actions]- The actions that can be performed on the account.
Child Properties
Optional Properties
id
stringThe ID of the action.type
enumAn enum representing the possible actions that can be performed on a PayLink Account. This comprehensive list includes all actions available for a company. However, each user will only see a subset of these actions, tailored to their specific account and plan.change-plan
cancel-plan
pause-plan
view-account
refresh
automated
booleanWhether or not the action is automated. Iftrue
, a task will be created to track and peform the automation. The status of the task can be monitorieed using webhooks. Iffalse
, then the user will perform the action on their device.
Optional Properties
monthlySavings
numberThe projected monthly savings if the user were to take the recommended action.category
enumAn enum of the types of categories of suggetionschange-plan
cancel-plan
downgrade-plan
remove-service
common-cancellation
redundant-services
costly-feature
alternative-service
underutilization
high-interest-rate
shortDescription
stringA short description of the suggestion.detailedDescription
stringA longer, more detailed description of the suggestion.buttonTitle
stringA short string intended to be used as the CTA in a button for this suggestion.identifier
stringA base64 encoded string that serves as the identifier for the suggestion.
{
"accounts": [
{
"_id": "67376c5befe988922e8aabc4",
"company": {
"_id": "6529a6eccb8e7200085c6ef6",
"name": "Hulu",
"branding": {
"logo": {
"url": "https://cdn-public.atomicfi.com/60e83c17-cda4-4b5a-98c6-c83199c54d48.png",
"backgroundColor": "#040405"
},
"color": "#040405"
}
},
"type": "linked",
"status": "connected",
"processing": false,
"name": "Hulu",
"account": {
"sourceId": "70236f02-dc3e-3419-bbf7-06d3da9d6685",
"type": "subscription",
"identities": [
{
"firstName": "test",
"email": "test@email.com",
"phone": "5558675309"
}
],
"billing": {
"accountNumber": "8498390016491919",
"amount": 20.46,
"dueDate": "2024-11-20T23:59:59.000Z",
"autopay": {
"status": "enabled",
"cycle": "monthly"
},
"items": [
{
"description": "Gibabit internet",
"amount": 76.99
},
{
"description": "Pay per view",
"amount": 45.89
}
],
"history": [
{
"date": "2024-10-20T18:00:54.761Z",
"amount": 20.7
},
{
"date": "2024-09-20T09:48:31.093Z",
"amount": 19.38
}
],
"paymentMethods": [
{
"type": "card",
"lastFour": "2345",
"brand": "Mastercard",
"isPrimary": true
}
]
},
"data": {
"plans": [
{
"sourceId": "Hulu (No Ads)",
"status": "active",
"title": "Family Hulu Account",
"description": "Hulu (No Ads)",
"freeTrial": false
}
],
"usage": [
{
"title": "profile",
"description": "Test Profile"
}
]
},
"_id": "67376c5befe988922e8aabc4"
},
"suggestions": [
{
"monthlySavings": 10,
"category": "remove-service",
"shortDescription": "Remove Disney Bundle",
"detailedDescription": "You are currently paying $10 per month for the Disney Bundle. If you are not using this service, consider removing it to save $10 monthly.",
"buttonTitle": "Remove Now",
"identifier": "BASE64_ENCODED_ID",
"action": {
"type": "cancel-plan",
"id": "BASE64_ENCODED_ID",
"automated": true
}
},
{
"monthlySavings": 5,
"category": "underutilization",
"shortDescription": "Switch to 5G Start Plan",
"detailedDescription": "Your line is on the 5G Start plan with a $3 rate adjustment. Consider switching to a plan without the adjustment or a lower-tier plan if the current plan features are underutilized.",
"buttonTitle": "Switch Plan",
"action": {
"type": "change-plan",
"id": "BASE64_ENCODED_ID",
"automated": false
}
}
],
"actions": [
{
"type": "change-plan",
"id": "BASE64_ENCODED_ID",
"automated": false
},
{
"type": "cancel-plan",
"id": "BASE64_ENCODED_ID",
"automated": true
},
{
"type": "pause-plan",
"id": "BASE64_ENCODED_ID",
"automated": true
}
]
}
]
}
Get Account
This endpoint returns an account.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
GET
/pay-link/accounts/:idResponse
The response includes an Account
object.
account
object- An
Account
object.Child Properties
Required Properties
_id
string- Unique identifier for the PayLink Account connected by the end user.
company
Company- An object containing details of the company to which the PayLink Account is connected.
Child Properties
Optional Properties
_id
stringUnique identifier for the company.name
stringThe name of the company.branding
objectThe branding information of the company. Useful if you are surfacing logos in your experience.color
stringThe color of the company. Atomic uses this color to style the experience - buttons and other UI elements. status
enum- The status of the account, either
initial
,connected
, ordisconnected
. If the account isinitial
, then the account has been selected but the user has not attempted to connect to it. If the account isconnected
then the account can be accessed. If the account isdisconnected
, then we cannot access the account with the given credentials. processing
boolean- A boolean value indicating whether or not the account is processing.
name
string- A name that can be used to display the account to the user. This field will be selected based of off the availablilty of data. In most cases it will either be
account.name
orcompany.name
. In cases where we cannot identify an appropriate name, it will be the stringUnknown Expense
. type
enum- An enum of the status of the account. Options include:
linked
,suggested
andunsupported
. Iflinked
, then the user has logged into the account and the user's data has been retrieved. Ifsuggested
, then the account has either been selected by the user or by our automated detection engine, but the user has not logged in yet. Ifunsupported
, then the account has been detected by our automation engine, but Atomic does not currently support integration for the account. account
Account- An object containing specific details of the account connected by the end user.
Child Properties
Required Properties
sourceId
string- An ID of the account pulled from the connected system.
type
enum- An enum of the type of account. Options include:
subscription
loan
policy
telecom
Optional Properties
name
stringThe name of the account.identities
[Identity]The identities connected to the account.Child Properties
Optional Properties
firstName
stringThe first name of the identity.lastName
stringThe last name of the identity.email
stringThe email associated with the identity.phone
stringThe phone number associated with the identity.address
stringThe address associated with the identity.address2
stringThe line 2 of the address associated with the identity.city
stringThe city of the address associated with the identity.state
stringThe state of the address associated with the identity.postalCode
stringThe postalCode of the address associated with the identity.billing
objectAn object containing the billing details of the account.Child Properties
Optional Properties
accountNumber
stringThe account number associated with the billing details for this account.amount
numberThe amount of the bill.dueDate
stringThe upcoming due date for the bill.autopay
objectitems
[BillingItems]history
[BillingHistory]paymentMethods
[PaymentMethod]An array of objects containing the payment methods associated with the account.Child Properties
Optional Properties
type
enumThe type of the payment method. One ofbank
,card
orpaypal
.lastFour
stringThe last four digits of the payment method.brand
stringThe brand of the payment method.isPrimary
booleanWhether the payment method is the primary payment method.description
stringThe description of the payment method.expiry
dateThe date the payment method expires.accountNumber
stringThe account number of the payment method. Only applicable fortype
ofbank
.routingNumber
stringThe routing number of the payment method. Only applicable fortype
ofbank
.data
objectThis object is dynamic based on the accounttype
.Child Properties
Optional Properties
plans
[Plan]The plans associated with the account. This field will be included if the accounttype
is eithersubscription
ortelecom
.Child Properties
Optional Properties
sourceId
stringThe source ID of the plan.status
stringThe status of the plan.title
stringThe title of the plan.description
stringThe description of the plan.freeTrial
booleanWhether the plan is on a free trial.usage
[Usage]The usage of the account. This field will be included if the accounttype
is eithersubscription
ortelecom
.Child Properties
Optional Properties
title
stringThe title of the usage.description
stringThe description of the usage.used
numberThe quantity of the plan used.limit
numberThe limit of the plan available to use.unit
stringThe units of usage of the plan. One ofgigabytes
,megabytes
,minutes
orkilowatts-per-hour
.lastUsed
dateThe last date the the plan was used. actions
[Actions]- The actions that can be performed on the account.
Child Properties
Optional Properties
id
stringThe ID of the action.type
enumAn enum representing the possible actions that can be performed on a PayLink Account. This comprehensive list includes all actions available for a company. However, each user will only see a subset of these actions, tailored to their specific account and plan.change-plan
cancel-plan
pause-plan
view-account
refresh
automated
booleanWhether or not the action is automated. Iftrue
, a task will be created to track and peform the automation. The status of the task can be monitorieed using webhooks. Iffalse
, then the user will perform the action on their device.
Optional Properties
suggestions
[Suggestions]An array of suggestion objects containing information about suggestions Atomic recommends presenting to the user based on the state of their account in the connected systemChild Properties
Required Properties
actions
[Actions]- The actions that can be performed on the account.
Child Properties
Optional Properties
id
stringThe ID of the action.type
enumAn enum representing the possible actions that can be performed on a PayLink Account. This comprehensive list includes all actions available for a company. However, each user will only see a subset of these actions, tailored to their specific account and plan.change-plan
cancel-plan
pause-plan
view-account
refresh
automated
booleanWhether or not the action is automated. Iftrue
, a task will be created to track and peform the automation. The status of the task can be monitorieed using webhooks. Iffalse
, then the user will perform the action on their device.
Optional Properties
monthlySavings
numberThe projected monthly savings if the user were to take the recommended action.category
enumAn enum of the types of categories of suggetionschange-plan
cancel-plan
downgrade-plan
remove-service
common-cancellation
redundant-services
costly-feature
alternative-service
underutilization
high-interest-rate
shortDescription
stringA short description of the suggestion.detailedDescription
stringA longer, more detailed description of the suggestion.buttonTitle
stringA short string intended to be used as the CTA in a button for this suggestion.identifier
stringA base64 encoded string that serves as the identifier for the suggestion.
{
"account": {
"_id": "67376c5befe988922e8aabc4",
"company": {
"_id": "6529a6eccb8e7200085c6ef6",
"name": "Hulu",
"branding": {
"logo": {
"url": "https://cdn-public.atomicfi.com/60e83c17-cda4-4b5a-98c6-c83199c54d48.png",
"backgroundColor": "#040405"
},
"color": "#040405"
}
},
"type": "linked",
"status": "connected",
"processing": false,
"name": "Hulu",
"account": {
"sourceId": "70236f02-dc3e-3419-bbf7-06d3da9d6685",
"type": "subscription",
"identities": [
{
"firstName": "test",
"email": "test@email.com",
"phone": "5558675309"
}
],
"billing": {
"accountNumber": "8498390016491919",
"amount": 20.46,
"dueDate": "2024-11-20T23:59:59.000Z",
"autopay": {
"status": "enabled",
"cycle": "monthly"
},
"items": [
{
"description": "Gibabit internet",
"amount": 76.99
},
{
"description": "Pay per view",
"amount": 45.89
}
],
"history": [
{
"date": "2024-10-20T18:00:54.761Z",
"amount": 20.7
},
{
"date": "2024-09-20T09:48:31.093Z",
"amount": 19.38
}
],
"paymentMethods": [
{
"type": "card",
"lastFour": "2345",
"brand": "Mastercard",
"isPrimary": true
}
]
},
"data": {
"plans": [
{
"sourceId": "Hulu (No Ads)",
"status": "active",
"title": "Family Hulu Account",
"description": "Hulu (No Ads)",
"freeTrial": false
}
],
"usage": [
{
"title": "profile",
"description": "Test Profile"
}
]
},
"_id": "67376c5befe988922e8aabc4"
},
"suggestions": [
{
"monthlySavings": 10,
"category": "remove-service",
"shortDescription": "Remove Disney Bundle",
"detailedDescription": "You are currently paying $10 per month for the Disney Bundle. If you are not using this service, consider removing it to save $10 monthly.",
"buttonTitle": "Remove Now",
"identifier": "BASE64_ENCODED_ID",
"action": {
"type": "cancel-plan",
"id": "BASE64_ENCODED_ID",
"automated": true
}
},
{
"monthlySavings": 5,
"category": "underutilization",
"shortDescription": "Switch to 5G Start Plan",
"detailedDescription": "Your line is on the 5G Start plan with a $3 rate adjustment. Consider switching to a plan without the adjustment or a lower-tier plan if the current plan features are underutilized.",
"buttonTitle": "Switch Plan",
"action": {
"type": "change-plan",
"id": "BASE64_ENCODED_ID",
"automated": false
}
}
],
"actions": [
{
"type": "change-plan",
"id": "BASE64_ENCODED_ID",
"automated": false
},
{
"type": "cancel-plan",
"id": "BASE64_ENCODED_ID",
"automated": true
},
{
"type": "pause-plan",
"id": "BASE64_ENCODED_ID",
"automated": true
}
]
}
}
Detect Accounts
This endpoint accepts transaction data for a specific end user. Atomic uses this data to identify recurring payments and recommend companies for the user to connect to and manage via our APIs.
The response returns an array of Account objects, providing details on whether the detected merchants are supported by Atomic along with other relevant information.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
Required Properties
financialTransactions
[FinancialTransactions]- An array of financial transaction objects.
Child Properties
Required Properties
id
string- An ID of the transaction.
date
string- The date the transation was processed, in ISO8601 format.
description
string- The transaction description from the merchant.
amount
number- Amount of the transaction.
Optional Properties
currency
enumCurrency in which the transaction was processed.usd
eur
cad
merchantName
stringThe name of the merchant associated with the transaction.type
enumAn enum of the type of transaction. Options are:category
stringCategory of the transaction.subCategory
stringOptional subcategory of the transaction.
POST
/pay-link/accountsResponse
The response contains an array of Account
objects containing and relevant metadata relating to the Accounts connected by the end user.
accounts
[Account]- An array of
Account
objects.Child Properties
Required Properties
_id
string- Unique identifier for the PayLink Account connected by the end user.
company
Company- An object containing details of the company to which the PayLink Account is connected.
Child Properties
Optional Properties
_id
stringUnique identifier for the company.name
stringThe name of the company.branding
objectThe branding information of the company. Useful if you are surfacing logos in your experience.color
stringThe color of the company. Atomic uses this color to style the experience - buttons and other UI elements. status
enum- The status of the account, either
initial
,connected
, ordisconnected
. If the account isinitial
, then the account has been selected but the user has not attempted to connect to it. If the account isconnected
then the account can be accessed. If the account isdisconnected
, then we cannot access the account with the given credentials. processing
boolean- A boolean value indicating whether or not the account is processing.
name
string- A name that can be used to display the account to the user. This field will be selected based of off the availablilty of data. In most cases it will either be
account.name
orcompany.name
. In cases where we cannot identify an appropriate name, it will be the stringUnknown Expense
. type
enum- An enum of the status of the account. Options include:
linked
,suggested
andunsupported
. Iflinked
, then the user has logged into the account and the user's data has been retrieved. Ifsuggested
, then the account has either been selected by the user or by our automated detection engine, but the user has not logged in yet. Ifunsupported
, then the account has been detected by our automation engine, but Atomic does not currently support integration for the account. account
Account- An object containing specific details of the account connected by the end user.
Child Properties
Required Properties
sourceId
string- An ID of the account pulled from the connected system.
type
enum- An enum of the type of account. Options include:
subscription
loan
policy
telecom
Optional Properties
name
stringThe name of the account.identities
[Identity]The identities connected to the account.Child Properties
Optional Properties
firstName
stringThe first name of the identity.lastName
stringThe last name of the identity.email
stringThe email associated with the identity.phone
stringThe phone number associated with the identity.address
stringThe address associated with the identity.address2
stringThe line 2 of the address associated with the identity.city
stringThe city of the address associated with the identity.state
stringThe state of the address associated with the identity.postalCode
stringThe postalCode of the address associated with the identity.billing
objectAn object containing the billing details of the account.Child Properties
Optional Properties
accountNumber
stringThe account number associated with the billing details for this account.amount
numberThe amount of the bill.dueDate
stringThe upcoming due date for the bill.autopay
objectitems
[BillingItems]history
[BillingHistory]paymentMethods
[PaymentMethod]An array of objects containing the payment methods associated with the account.Child Properties
Optional Properties
type
enumThe type of the payment method. One ofbank
,card
orpaypal
.lastFour
stringThe last four digits of the payment method.brand
stringThe brand of the payment method.isPrimary
booleanWhether the payment method is the primary payment method.description
stringThe description of the payment method.expiry
dateThe date the payment method expires.accountNumber
stringThe account number of the payment method. Only applicable fortype
ofbank
.routingNumber
stringThe routing number of the payment method. Only applicable fortype
ofbank
.data
objectThis object is dynamic based on the accounttype
.Child Properties
Optional Properties
plans
[Plan]The plans associated with the account. This field will be included if the accounttype
is eithersubscription
ortelecom
.Child Properties
Optional Properties
sourceId
stringThe source ID of the plan.status
stringThe status of the plan.title
stringThe title of the plan.description
stringThe description of the plan.freeTrial
booleanWhether the plan is on a free trial.usage
[Usage]The usage of the account. This field will be included if the accounttype
is eithersubscription
ortelecom
.Child Properties
Optional Properties
title
stringThe title of the usage.description
stringThe description of the usage.used
numberThe quantity of the plan used.limit
numberThe limit of the plan available to use.unit
stringThe units of usage of the plan. One ofgigabytes
,megabytes
,minutes
orkilowatts-per-hour
.lastUsed
dateThe last date the the plan was used. actions
[Actions]- The actions that can be performed on the account.
Child Properties
Optional Properties
id
stringThe ID of the action.type
enumAn enum representing the possible actions that can be performed on a PayLink Account. This comprehensive list includes all actions available for a company. However, each user will only see a subset of these actions, tailored to their specific account and plan.change-plan
cancel-plan
pause-plan
view-account
refresh
automated
booleanWhether or not the action is automated. Iftrue
, a task will be created to track and peform the automation. The status of the task can be monitorieed using webhooks. Iffalse
, then the user will perform the action on their device.
Optional Properties
suggestions
[Suggestions]An array of suggestion objects containing information about suggestions Atomic recommends presenting to the user based on the state of their account in the connected systemChild Properties
Required Properties
actions
[Actions]- The actions that can be performed on the account.
Child Properties
Optional Properties
id
stringThe ID of the action.type
enumAn enum representing the possible actions that can be performed on a PayLink Account. This comprehensive list includes all actions available for a company. However, each user will only see a subset of these actions, tailored to their specific account and plan.change-plan
cancel-plan
pause-plan
view-account
refresh
automated
booleanWhether or not the action is automated. Iftrue
, a task will be created to track and peform the automation. The status of the task can be monitorieed using webhooks. Iffalse
, then the user will perform the action on their device.
Optional Properties
monthlySavings
numberThe projected monthly savings if the user were to take the recommended action.category
enumAn enum of the types of categories of suggetionschange-plan
cancel-plan
downgrade-plan
remove-service
common-cancellation
redundant-services
costly-feature
alternative-service
underutilization
high-interest-rate
shortDescription
stringA short description of the suggestion.detailedDescription
stringA longer, more detailed description of the suggestion.buttonTitle
stringA short string intended to be used as the CTA in a button for this suggestion.identifier
stringA base64 encoded string that serves as the identifier for the suggestion.
{
"accounts": [
{
"_id": "67376c5befe988922e8aabc4",
"company": {
"_id": "6529a6eccb8e7200085c6ef6",
"name": "Hulu",
"branding": {
"logo": {
"url": "https://cdn-public.atomicfi.com/60e83c17-cda4-4b5a-98c6-c83199c54d48.png",
"backgroundColor": "#040405"
},
"color": "#040405"
}
},
"type": "linked",
"status": "connected",
"processing": false,
"name": "Hulu",
"account": {
"sourceId": "70236f02-dc3e-3419-bbf7-06d3da9d6685",
"type": "subscription",
"identities": [
{
"firstName": "test",
"email": "test@email.com",
"phone": "5558675309"
}
],
"billing": {
"accountNumber": "8498390016491919",
"amount": 20.46,
"dueDate": "2024-11-20T23:59:59.000Z",
"autopay": {
"status": "enabled",
"cycle": "monthly"
},
"items": [
{
"description": "Gibabit internet",
"amount": 76.99
},
{
"description": "Pay per view",
"amount": 45.89
}
],
"history": [
{
"date": "2024-10-20T18:00:54.761Z",
"amount": 20.7
},
{
"date": "2024-09-20T09:48:31.093Z",
"amount": 19.38
}
],
"paymentMethods": [
{
"type": "card",
"lastFour": "2345",
"brand": "Mastercard",
"isPrimary": true
}
]
},
"data": {
"plans": [
{
"sourceId": "Hulu (No Ads)",
"status": "active",
"title": "Family Hulu Account",
"description": "Hulu (No Ads)",
"freeTrial": false
}
],
"usage": [
{
"title": "profile",
"description": "Test Profile"
}
]
},
"_id": "67376c5befe988922e8aabc4"
},
"suggestions": [
{
"monthlySavings": 10,
"category": "remove-service",
"shortDescription": "Remove Disney Bundle",
"detailedDescription": "You are currently paying $10 per month for the Disney Bundle. If you are not using this service, consider removing it to save $10 monthly.",
"buttonTitle": "Remove Now",
"identifier": "BASE64_ENCODED_ID",
"action": {
"type": "cancel-plan",
"id": "BASE64_ENCODED_ID",
"automated": true
}
},
{
"monthlySavings": 5,
"category": "underutilization",
"shortDescription": "Switch to 5G Start Plan",
"detailedDescription": "Your line is on the 5G Start plan with a $3 rate adjustment. Consider switching to a plan without the adjustment or a lower-tier plan if the current plan features are underutilized.",
"buttonTitle": "Switch Plan",
"action": {
"type": "change-plan",
"id": "BASE64_ENCODED_ID",
"automated": false
}
}
],
"actions": [
{
"type": "change-plan",
"id": "BASE64_ENCODED_ID",
"automated": false
},
{
"type": "cancel-plan",
"id": "BASE64_ENCODED_ID",
"automated": true
},
{
"type": "pause-plan",
"id": "BASE64_ENCODED_ID",
"automated": true
}
]
}
]
}
Delete Accounts
This endpoint will delete all accounts for the specified user.
A successful response will return a JSON object with a result object containing success
equal to true
.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
DELETE
/pay-link/accounts/Delete Account
This endpoint will delete an account.
A successful response will return a JSON object with a result object containing success
equal to true
.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
DELETE
/pay-link/accounts/:idSuggestions
An Suggestion
in the Atomic context refers to a document that represents a possible method that a user could use to save money on their bill.
Review a Suggestion
This endpoint is used to review a Suggestion
. Reviews are used to improve our suggestion generation engine. Suggestions reviewed with the rating of not-interested
will be filtered out when accounts
are returned via webhooks and api endpoints. Currently, only the rating value not-interested
is supported.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
POST
/pay-link/suggestion-reviewTask
Each discrete operation processed through the Atomic system, such as an update to a payment account on file or an action against a merchant system on behalf of a user, will instantiate what is referred to as a Task. A Task consists of an authentication step where Atomic logs into merchant system or service provider and an operation step either data is read or an update is written to the system in question.
Get Task Details
This endpoint returns the details of a Task. Submit the corresponding taskId
and receive the details of that Task.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
Required Properties
taskId
string- The id of the Task. The
taskId
property is sent in multiple webhooks or any of the interaction events which are fired after the authentication phase of the flow has begun.
GET
/task/:taskId/detailsResponse
A successful request will return the details of the Task.
_id
string- The id of the Task.
authenticated
boolean- Indicates whether or not the Task has successfully authenticated against the merchant system.
company
object- Metadata for the employer.
Child Properties
connector
object- Metadata for the connector.
Child Properties
createdAt
date- The timestamp when the task was created, stored in UTC and ISO 8601 format.
updatedAt
date- The timestamp when the task was last updated, stored in UTC and ISO 8601 format.
failReason
stringOptional- The failure reason associated with a failed task. Will consist of one of the failure values.
linkedAccount
stringOptional- The id of the linked account used to create the task.
metadata
objectOptional- The
metadata
provided by your system when the task was initialized. product
string- The product of the task. Possible values include
present
andswitch
. status
string- The status of the task which may be
queued
,processing
,failed
, orcompleted
. taskWorkflow
object- The Task Workflow of the Task. When multiple tasks are associated with a single workflow, they will all have the same
taskWorkflow
object. Every Task is associated with a Task Workflow, even if it not associated with another Task. user
object- The user who processed the Task.
Child Properties
Required Properties
_id
string- The id of the user in atomic's system.
identifier
string- The user's unique identifier, as sent to Atomic during AccessToken creation.
{
"data": {
"task": {
"_id": "65b15fd99b3f03aa9edff9de",
"authenticated": true,
"company": {
"_id": "607e249736b9f053b536bde0",
"branding": {
"logo": {
"url": "https://cdn-public.atomicfi.com/b02670fa-ce1b-4171-855b-6856b37bb938.png"
}
},
"name": "Test Company"
},
"connector": {
"_id": "607e249736b9f053b536bde1",
"branding": {
"logo": {
"url": "https://cdn-public.atomicfi.com/b02670fa-ce1b-4171-855b-6856b37bb938.png"
}
},
"name": "Test Connector"
},
"createdAt": "2024-01-01T00:00:00.000Z",
"updatedAt": "2024-01-01T00:00:05.000Z",
"failReason": "unknown-failure",
"linkedAccount": "645963a0b754649972f3d4ac",
"metadata": {
"data": "test"
},
"product": "switch",
"status": "completed",
"taskWorkflow": {
"_id": "643f2ed34253e21bdbd2de31"
},
"user": {
"_id": "607e249736b9f053b536bdea",
"identifier": "IDENTIFIER_FROM_YOUR_SYSTEM"
}
}
}
}
Get Task Status
Calling this API returns the status of a Task. Submit the corresponding taskId
and receive the status
of that Task.
This endpoint is designed to be lightweight and intended for polling use cases.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
Required Properties
taskId
string- The id of the Task. The
taskId
property is sent in multiple webhooks or any of the interaction events which are fired after the authentication phase of the flow has begun.
GET
/task/:taskId/statusResponse
A successful request will return the createdAt
date, status
, and if a Task failed (Tasks with a status of "failed") a failReason
.
createdAt
date- The timestamp when the Task was created, stored in UTC and ISO 8601 format.
status
string- The status of the task which may be
queued
,processing
,failed
, orcompleted
. failReason
stringOptional- The failure reason associated with a failed task. Will consist of one of the failure values.
{
"createdAt": "2023-01-03T16:40:48.009Z",
"status": "failed",
"failReason": "payment-method-not-supported"
}
User
A User
is an entity in the Atomic system that is created during the access token creation process. Details about a user's accounts are stored on the user object for reference as needed in an operation; ie. for adding a deposit account to a payroll system or updating the card-on-file for a merchant service.
End Monitoring
This endpoint is used to remove monitoring for users that have payroll data monitoring enabled via continuous access.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
Required Properties
identifier
string- The user's unique identifier, as sent to Atomic during AccessToken creation.
PUT
/user/:identifier/end-monitoring{
"success": true
}
Get Tasks
This endpoint is used to retrieve tasks for the specified user.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
Required Properties
identifier
string- The user's unique identifier, as sent to Atomic during AccessToken creation.
Optional Properties
status
string
queued
, processing
, failed
, or completed
.payrollDataAvailable
string
GET
/user/:identifier/tasksResponse
A successful response will return an object with data.tasks
equal to an array of task objects with the following properties.
_id
string- The id of the task.
authenticated
boolean- A boolean value indication whether or not the task has successfully authenticated against the payroll system.
createdAt
date- The timestamp when the task was created, stored in UTC and ISO 8601 format.
failReason
stringOptional- The failure reason associated with a failed task. Will consist of one of the failure values.
payrollDataAvailable
boolean- A boolean value indicating whether or not the user's payroll data is ready for retrieval via api.
product
enum- The product relevant to the executed task. Options are:
deposit
verify
status
string- The status of the task which may be
queued
,processing
,failed
, orcompleted
.
{
"data": {
"tasks": [
{
"_id": "65b3f3c8b871a9626c36d103",
"authenticated": true,
"createdAt": "2024-01-26T18:02:55.795Z",
"payrollDataAvailable": true,
"product": "verify",
"status": "completed"
},
{
"_id": "65b3f3c8b871a9626c36d103",
"authenticated": true,
"createdAt": "2024-01-26T18:02:55.795Z",
"failReason": "bad-credentials",
"payrollDataAvailable": false,
"product": "deposit",
"status": "failed"
}
]
}
}
Update User
This endpoint is used to add data to a user in response to an onDataRequest
event emitted from our Transact SDK. Any data passed will be upserted into the user's object. The typical use case is to delay the transmission of sensitive data (bank accounts, cards, identity information, etc.) until our system has need for such data.
The shape of the request body is similar to our access token creation endpoint, except the data to be added/updated will be nested in a data
key, and the identifier
will not be nested, but remain on the first-level of the object.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
PUT
/user