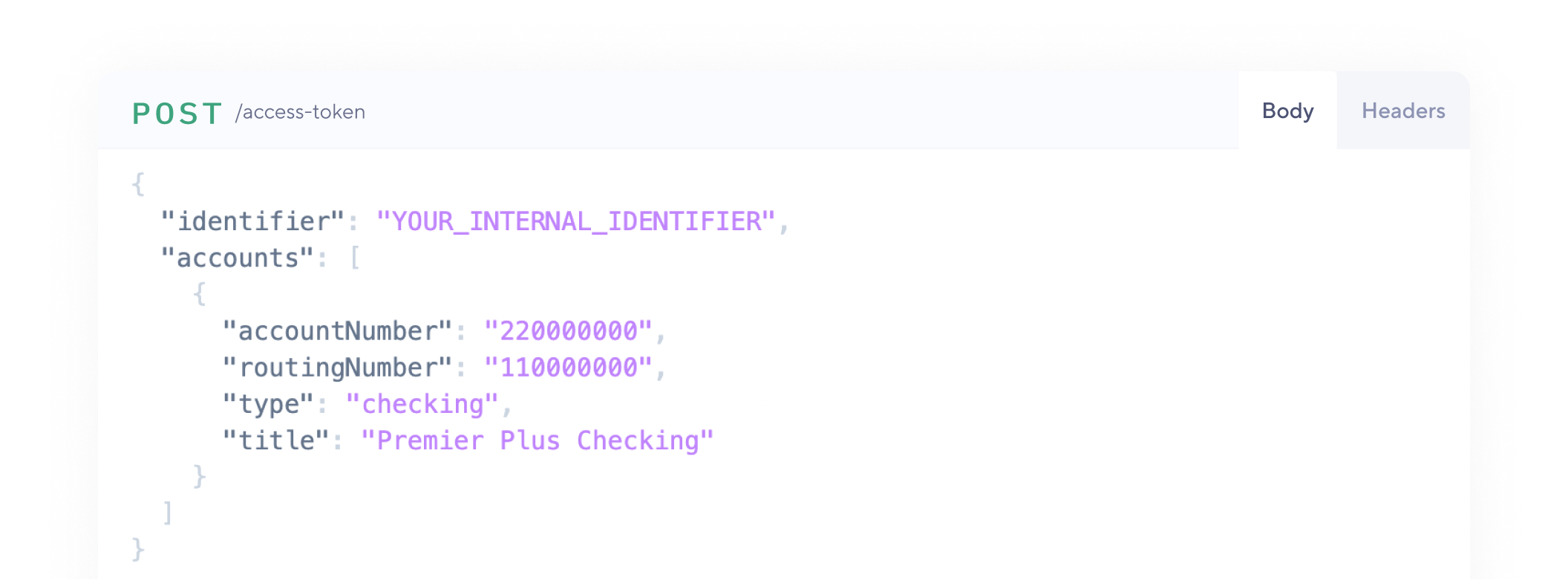
UserLink API Reference
Atomic's API is built around RESTful principles, using JSON encoded request and response bodies.
To get going quickly, we recommend using Postman - an API collaboration tool. Atomic provides a public workspace which you can fork into your own workspace. Use the button below to get started.
The workspace comes fully documented with:
- several useful flows using our APIs
- requests for all of the Atomic endpoints
- an environment containing placeholders for all the variables needed to connect to your Sandbox instance of Console
Authentication
Atomic uses a combination of API keys and access tokens to authenticate requests. Your API keys carry many privileges, so be sure to keep them secure! Do not share your secret API keys in publicly accessible areas such as GitHub, client-side code, etc.
API Key and Secret
You can retrieve and manage your API key and secrets on the credentials page on the Atomic Console. Include these headers in requests that are secured with your API Key and Secret:
Header | Description |
---|---|
x-api-key | API Key for your Atomic account |
x-api-secret | API Secret for your Atomic account |
Access Token
A publicToken
will be included in the headers of requests which are made via the end user from client-side code, e.g. searching for their employer or initializing a Task. The publicToken
defaults to a 24 hour expiry, but can be set to a minimum of 30 minutes or a maximum of 30 days by passing in the desired duration using the optional tokenLifetime
property in the call to /access-token
. The minimum is to allow for users to complete variations of the flows, such as MFA, which can take time.
Header | Description |
---|---|
x-public-token | Public token generated during access token creation. |
Errors
Our API returns standard HTTP success or error status codes. For errors, we will also include extra information about what went wrong encoded in the response as JSON. The various HTTP status codes we might return are listed below.
Code | Title | Description |
---|---|---|
200 | OK | The request was successful. |
400 | Bad Request | The request was unacceptable. |
401 | Unauthorized | Missing or invalid credentials. |
404 | Not Found | The resource does not exist. |
422 | Validation Error | A validation error occurred. |
429 | Too Many Requests | Too may requests, please try again later. |
50X | Internal Server Error | An error occurred with our API. |
Access Token
An AccessToken
grants access to Atomic's API resources for an end user when its value is used as the x-public-token
header.
Create Access Token
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
Required Properties
identifier
string- A unique identifier from your system that will be used to reference this user. This could be a primary key used in your system or another unique identifier. Creating multiple access tokens with the same identifier will tie those tokens to the same user in Atomic's system.
POST
/access-tokenProduct Specific Properties
The identifier
property is required for generating all AccessTokens
, but each Atomic product may require some additional context in order to correctly set up the flow in our backend. Please refer to the implementation pages for each Product to get specific details regarding AccessToken
creation.
Response
Successfully creating an Access Token will return a payload with a data object containing a publicToken
. The publicToken
may be used to initialize the Transact SDK and is valid for tokenLifetime
seconds.
{
"data": {
"publicToken": "\"PUBLIC_TOKEN\""
}
}
Revoke Access Token
This endpoint is used to revoke an existing AccessToken
. This action invalidates the token, preventing it from being used to access Atomic's API resources.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
PUT
/access-token/:publicToken/revokeCoAuth
Atomic has established direct partnerships with select payroll systems to enable seamless user lookups, a capability branded as CoAuth.
By identifying a user and leveraging the payroll system’s stored multi-factor authentication (MFA) flow, Atomic can securely verify the user’s identity. Once verified, the payroll system provides the necessary access for Atomic to update the user’s direct deposit information efficiently; reducing friction, enhancing conversion rates, and overall improving the user experience.
Pre-screen
The Pre-Screen API allows for pre-screening a user through CoAuth by using their identity data to perform a lookup within supported payroll systems. This API is typically called after the user expresses intent to set up direct deposit but before launching them into Transact.
Once the user is identified within a payroll system, Atomic can seamlessly set up direct deposit on their behalf, improving conversion rates and creating a more streamlined user experience.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
Required Properties
identifier
string- The user's unique identifier, to be used again during AccessToken creation.
identity
string- An object containing identity data for the user to be used by Atomic for the lookup operation in the CoAuth enabled payroll system.
POST
/pre-screenResponse
A successful pre-screen will return an array of IncomeSources
. Each object in the array will contain details about the payroll system where the user was verified and a data object with specifics regarding the lookup operation.
incomeSources
[IncomeSource]- An array of IncomeSource objects containing details of the lookups performed by Atomic in the CoAuth enabled payroll systems.
Child Properties
Required Properties
data
object- An object with details about the lookup operation in the payroll system.
Child Properties
Required Properties
userStatus
enum- The status of the lookup. Options are:
VERIFIED_PAYROLL
NOT_FOUND
UNSUPPORTED_PAYROLL
origin
enum- The origin of the income source verification. Currentyl limited to
pre-screen
. lookupDate
string- The timestamp of when the income source was derived or verified. Returned in ISO 8601 format.
{
"incomeSources": [
{
"data": {
"userStatus": "VERIFIED_PAYROLL",
"origin": "pre-screen",
"lookupDate": "2024-08-27T16:44:21.482Z"
},
"company": {
"_id": "5d38f1e8512bbf71fb776015",
"name": "ADP",
"branding": {
"logo": {
"url": "https://atomicfi-public-production.s3.amazonaws.com/a8d7e778-b718-45e0-b639-2305e33e7f95_ADP.png",
"backgroundColor": "#F2F2F2"
},
"color": "#9460FE"
}
}
}
]
}
Company
A user has the option to link their payroll through their payroll provider or by navigating our employer-to-payroll system mapping. We have the notion of a Company that encompasses both aspects.
Company Search
Use this endpoint to build a typeahead experience, as employed in our Transact SDK, or to get the company's _id
for deeplinking to the login section of Transact.
Search for a Company using a text query
. Searches can also be filtered by passing in additional properties detailed below.
Authentication can be handled via either the API Key and Secret method or the Access Token method.
Optional Properties
scopes
enum
property
. Options are: pay-link
user-link
product
string
deposit
, verify
, and tax
.products
[string]
deposit
, verify
, and tax
.tags
[string]
gig-economy
, payroll-provider
, and unemployment
.excludedTags
[string]
gig-economy
, payroll-provider
, and unemployment
.POST
/company/searchResponse
Successfully querying the Company search endpoint will return a payload with a data
array of Company objects. When a company is under-maintenance
, users are unable to initiate an authentication until it has been restored.
_id
string- Unique identifier for the company.
name
string- Company name.
status
string- Possible values include
operational
orunder-maintenance
. connector.availableProducts
[string]- A list of compatible products.
branding.logo.url
string- Logo for the company, in PNG format.
branding.color
string- Branding color for the company.
tags
[string]- Tags with which a company is associated. Possible values include
gig-economy
,payroll-provider
,unemployment
, andmanual-deposits
.
{
"data": [
{
"_id": "5d38f1e8512bbf71fb776015",
"name": "DoorDash",
"status": "operational",
"connector": {
"_id": "5d38f182512bbf0c06776013",
"availableProducts": [
"deposit"
],
"capabilities": {
"distributionTypes": [
"total"
]
}
},
"branding": {
"logo": {
"url": "https://atomicfi-public-production.s3.amazonaws.com/a8d7e778-b718-45e0-b639-2305e33e7f95_ADP.svg",
"backgroundColor": "#F2F2F2"
},
"color": "#9460FE"
},
"tags": [
"gig-economy"
]
}
]
}
Task
Each discrete payroll operation, such as a verification of income, direct deposit switch, or update to a payment account of file, will instantiate what is referred to as a Task in our system. A Task consists of an authentication step where Atomic logs into a payroll or service provider and an operation step where we read data or write to the system; either a direct deposit allocation or updating a payment account on file, depending on the Task.
Get Task Details
This endpoint returns the details of a Task. Submit the corresponding taskId
and receive the details of that Task.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
Required Properties
taskId
string- The id of the Task. The
taskId
property is sent in multiple webhooks or any of the interaction events which are fired after the authentication phase of the flow has begun.
GET
/task/:taskId/detailsResponse
A successful request will return the details of the task.
_id
string- The id of the task.
authenticated
boolean- Indicates whether or not the task has successfully authenticated against the payroll system.
company
object- Metadata for the employer.
Child Properties
connector
object- Metadata for the connector.
Child Properties
createdAt
date- The timestamp when the task was created, stored in UTC and ISO 8601 format.
updatedAt
date- The timestamp when the task was last updated, stored in UTC and ISO 8601 format.
depositData
objectOptional- If the task is a deposit task, this data will indicate the type of deposit switch performed.
Child Properties
Optional Properties
accountType
stringThe type of the account. Possible values includesavings
,checking
, orpaycard
.distributionAmount
numberThe amount being distributed to the account. WhendistributionType
ispercent
, the number represents a percentage of the total pay. WhendistributionType
isfixed
, this number represents a fixed dollar amount. This value is not set whendistributionType
istotal
.distributionType
stringThe type of distribution for the account. Possible values includetotal
,percent
, orfixed
.lastFour
stringThe last digits of the account number.routingNumber
stringThe routing number.title
stringThe title of the account. failReason
stringOptional- The failure reason associated with a failed task. Will consist of one of the failure values.
linkedAccount
stringOptional- The id of the linked account used to create the task.
metadata
objectOptional- The
metadata
provided by your system when the task was initialized. payrollDataAvailable
boolean- A boolean value indicating whether or not the user's payroll data is ready for retrieval via api.
product
string- The product of the task. Possible values include
deposit
,verify
, andtax
. status
string- The status of the task which may be
queued
,processing
,failed
, orcompleted
. taskWorkflow
object- The Task Workflow of the Task. When multiple tasks are associated with a single workflow, they will all have the same
taskWorkflow
object. Every Task is associated with a Task Workflow, even if it not associated with another Task. user
object- The user who processed the Task.
Child Properties
Required Properties
_id
string- The id of the user in atomic's system.
identifier
string- The user's unique identifier, as sent to Atomic during AccessToken creation.
{
"data": {
"task": {
"_id": "65b15fd99b3f03aa9edff9de",
"authenticated": true,
"company": {
"_id": "607e249736b9f053b536bde0",
"branding": {
"logo": {
"url": "https://cdn-public.atomicfi.com/b02670fa-ce1b-4171-855b-6856b37bb938.png"
}
},
"name": "Test Company"
},
"connector": {
"_id": "607e249736b9f053b536bde1",
"branding": {
"logo": {
"url": "https://cdn-public.atomicfi.com/b02670fa-ce1b-4171-855b-6856b37bb938.png"
}
},
"name": "Test Connector"
},
"createdAt": "2024-01-01T00:00:00.000Z",
"updatedAt": "2024-01-01T00:00:05.000Z",
"depositData": {
"accountType": "checking",
"distributionAmount": 10,
"distributionType": "fixed",
"lastFour": "0000",
"routingNumber": "22000000",
"title": "Checking Account"
},
"failReason": "unknown-failure",
"linkedAccount": "645963a0b754649972f3d4ac",
"metadata": {
"data": "test"
},
"payrollDataAvailable": false,
"product": "deposit",
"status": "failed",
"taskWorkflow": {
"_id": "643f2ed34253e21bdbd2de31"
},
"user": {
"_id": "607e249736b9f053b536bdea",
"identifier": "GUID"
}
}
}
}
Get Task Status
Calling this API returns the status of a Task. Submit the corresponding taskId
and receive the status
of that Task and payrollDataAvailable
which indicates that any fetchable data is ready to be pulled via the corresponding API calls.
This endpoint is designed to be lightweight and intended for polling use cases.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
Required Properties
taskId
string- The id of the Task. The
taskId
property is sent in multiple webhooks or any of the interaction events which are fired after the authentication phase of the flow has begun.
GET
/task/:taskId/statusResponse
A successful request will return the createdAt
date, status
, and if a Task failed (Tasks with a status of "failed") a failReason
.
createdAt
date- The timestamp when the Task was created, stored in UTC and ISO 8601 format.
status
string- The status of the task which may be
queued
,processing
,failed
, orcompleted
. payrollDataAvailable
boolean- A boolean value indicating whether or not the user's payroll data is ready for retrieval via api.
failReason
stringOptional- The failure reason associated with a failed task. Will consist of one of the failure values.
{
"data": {
"task": {
"createdAt": "2023-01-03T16:40:48.009Z",
"status": "failed",
"failReason": "no-data-found",
"payrollDataAvailable": false
}
}
}
Generate File URL
Generate a URL in order to download a user file (ex: paystubs PDFs). Each URL is valid for 1 hour.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
Required Properties
taskId
string- The
_id
of the Task, which can be retrieved either from interaction events during the Transact session with the user or from the associated webhooks. fileId
string- The
_id
of the file. This is returned in API calls for relevant data categories, such as/taxes
for W-2's, or sent via relevant webhooks when a file is retrieved from the payroll system.
GET
/task/:taskId/file/:fileId/generate-urlResponse
A successful request will return a URL that can be used to download the file.
url
string- A URL that can be used to download the file. The URL is valid for 1 hour.
{
"url": "[ATOMIC-GENERATED-PRESIGNED-S3-URL]"
}
PrescreenBeta
Prescreen is an endpoint you can call prior to task creation in order to get a predicted conversion confidence, offering additional insight into your user and the likelihood they will succeed using our service.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
POST
/task/prescreenResponse
The response returns an object with a single confidence property as a string value. We will make this determination based on a variety of proprietary considerations such as traffic volumes and calculations with other similar connector types.
confidence
string- The likelihood of the user being successful using our flow. Will return a value of "HIGH" or "LOW".
{
"data": {
"confidence": "HIGH"
}
}
Deposit Accounts
List deposit accounts once they've been synced to Atomic from the payroll provider.
For purely Deposit switch use cases, this information is not collected.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
Required Properties
identifier
string- The user's unique identifier, as sent to Atomic during AccessToken creation.
Optional Properties
linkedAccount
string
_id
of a LinkedAccount object provided as a query string parameter.task
string
_id
of the task, provided as a query string parameter, that was used to retrieve the data. If this parameter is not provided, the data that was most recently retrieved from the payroll system will be returned.includeOutputMetadata
string
true
, the outputMetadata
will be included in the response.GET
/deposit-accounts/:identifierResponse
A successful response will return all direct deposit accounts on file for the user.
data
[DepositAccounts]- An array of objects that include an array of deposit account objects,
company
,connector
,task
ID, andlinkedAccount
ID if enabled.
{
"data": [
{
"depositAccounts": [
[
{
"routingNumber": "123123123",
"accountNumber": "1122330000",
"type": "checking",
"bankName": "Molecular Bank",
"distributionType": "percent",
"distributionAmount": 80
},
{
"routingNumber": "456456456",
"accountNumber": "XXXX1111",
"type": "savings",
"bankName": "Molecular Bank",
"distributionType": "percent",
"distributionAmount": 20
}
]
],
"company": {
"_id": "5d77f9e1070856f3828945c6",
"name": "DoTerra",
"branding": {
"logo": {
"_id": "5eb62781b4b83f0008f638cc",
"url": "https://cdn.atomicfi.com/doterra-logo.svg"
}
}
},
"connector": {
"_id": "5d77f95207085632a58945c3",
"name": "ADP",
"branding": {
"logo": {
"_id": "5eb62781b4b83f0008f6343c",
"url": "https://cdn.atomicfi.com/adp-logo.svg"
}
}
},
"linkedAccount": "5f7212103a40e91f95ba376d",
"task": "602414d84f9a1980cf5eafcc"
}
]
}
Employment
Get employment data once its been synced to Atomic from the payroll provider.
For purely Deposit switch use cases, this information is not collected.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
Required Properties
identifier
string- The user's unique identifier, as sent to Atomic during AccessToken creation.
Optional Properties
linkedAccount
string
_id
of a LinkedAccount object provided as a query string parameter.task
string
_id
of the task, provided as a query string parameter, that was used to retrieve the data. If this parameter is not provided, the data that was most recently retrieved from the payroll system will be returned.includeOutputMetadata
string
true
, the outputMetadata
will be included in the response.GET
/employment/:identifierResponse
A successful request will return the employment data for the user.
data
[Employment]- An array of objects that include an employment object,
company
,connector
,task
ID, andlinkedAccount
ID if enabled.
{
"data": [
{
"employment": {
"employeeType": "fulltime",
"employmentStatus": "active",
"jobTitle": "Product Manager",
"startDate": "2017-04-19T12:00:00.000Z",
"minimumMonthsOfEmployment": 58,
"weeklyHours": 40,
"employer": {
"name": "Company Inc.",
"address": {
"line1": "12345 Enterprise Rd",
"line2": "Suite 105",
"city": "Salt Lake City",
"state": "UT",
"postalCode": "84111",
"country": "USA"
}
}
},
"company": {
"_id": "5d77f9e1070856f3828945c6",
"name": "DoTerra",
"branding": {
"logo": {
"_id": "5eb62781b4b83f0008f638cc",
"url": "https://cdn.atomicfi.com/doterra-logo.svg"
}
}
},
"connector": {
"_id": "5d77f95207085632a58945c3",
"name": "ADP",
"branding": {
"logo": {
"_id": "5eb62781b4b83f0008f6343c",
"url": "https://cdn.atomicfi.com/adp-logo.svg"
}
}
},
"linkedAccount": "5f7212103a40e91f95ba376d",
"task": "602414d84f9a1980cf5eafcc"
}
]
}
Identity
Get identity data once its been synced to Atomic from the payroll provider.
For purely Deposit switch use cases, this information is not collected.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
Required Properties
identifier
string- The user's unique identifier, as sent to Atomic during AccessToken creation.
Optional Properties
linkedAccount
string
_id
of a LinkedAccount object provided as a query string parameter.task
string
_id
of the task, provided as a query string parameter, that was used to retrieve the data. If this parameter is not provided, the data that was most recently retrieved from the payroll system will be returned.includeOutputMetadata
string
true
, the outputMetadata
will be included in the response.GET
/identity/:identifierResponse
A successful request will return the identity data for the user.
data
[Identity]- An array of objects that include an identity object,
company
,connector
,task
ID, andlinkedAccount
ID if enabled.
{
"data": [
{
"identity": {
"firstName": "Jane",
"lastName": "Appleseed",
"dateOfBirth": "1984-04-12T12:00:00.000Z",
"email": "janeappleseed@example.com",
"phone": "5558881111",
"ssn": "111223333",
"address": "123 Example St.",
"city": "Salt Lake City",
"state": "UT",
"postalCode": "84111"
},
"company": {
"_id": "5d77f9e1070856f3828945c6",
"name": "DoTerra",
"branding": {
"logo": {
"_id": "5eb62781b4b83f0008f638cc",
"url": "https://cdn.atomicfi.com/doterra-logo.svg"
}
}
},
"connector": {
"_id": "5d77f95207085632a58945c3",
"name": "ADP",
"branding": {
"logo": {
"_id": "5eb62781b4b83f0008f6343c",
"url": "https://cdn.atomicfi.com/adp-logo.svg"
}
}
},
"linkedAccount": "5f7212103a40e91f95ba376d",
"task": "602414d84f9a1980cf5eafcc"
}
]
}
Income
Get income data once its been synced to Atomic from the payroll provider.
For purely Deposit switch use cases, this information is not collected.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
Required Properties
identifier
string- The user's unique identifier, as sent to Atomic during AccessToken creation.
Optional Properties
linkedAccount
string
_id
of a LinkedAccount object provided as a query string parameter.task
string
_id
of the task, provided as a query string parameter, that was used to retrieve the data. If this parameter is not provided, the data that was most recently retrieved from the payroll system will be returned.includeOutputMetadata
string
true
, the outputMetadata
will be included in the response.GET
/income/:identifierResponse
A successful request will return the income data associated with the account.
data
[Income]- An array of objects that include an income object,
company
,connector
,task
ID, andlinkedAccount
ID if enabled.
{
"data": [
{
"income": {
"income": 45000,
"incomeType": "yearly",
"annualIncome": 45000,
"hourlyIncome": 21.56,
"netHourlyRate": 18.44,
"payCycle": "weekly",
"nextExpectedPayDate": "2020-06-30T12:00:00.000Z",
"currentPayPeriodStart": "2020-06-13T12:00:00.000Z",
"currentPayPeriodEnd": "2020-06-27T12:00:00.000Z",
"unpaidHoursInPayPeriod": 24
},
"company": {
"_id": "5d77f9e1070856f3828945c6",
"name": "DoTerra",
"branding": {
"logo": {
"_id": "5eb62781b4b83f0008f638cc",
"url": "https://cdn.atomicfi.com/doterra-logo.svg"
}
}
},
"connector": {
"_id": "5d77f95207085632a58945c3",
"name": "ADP",
"branding": {
"logo": {
"_id": "5eb62781b4b83f0008f6343c",
"url": "https://cdn.atomicfi.com/adp-logo.svg"
}
}
},
"linkedAccount": "5f7212103a40e91f95ba376d",
"task": "602414d84f9a1980cf5eafcc"
}
]
}
Statements
Get statements data once its been synced to Atomic from the payroll provider.
For purely Deposit switch use cases, this information is not collected.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
Required Properties
identifier
string- The user's unique identifier, as sent to Atomic during AccessToken creation.
Optional Properties
linkedAccount
string
_id
of a LinkedAccount object provided as a query string parameter.task
string
_id
of the task, provided as a query string parameter, that was used to retrieve the data. If this parameter is not provided, the data that was most recently retrieved from the payroll system will be returned.includeOutputMetadata
string
true
, the outputMetadata
will be included in the response.limit
string
GET
/statements/:identifierResponse
A successful request will return the statements for the user.
data
[Statement]- An array of objects that include a statements object,
company
,connector
,task
ID, andlinkedAccount
ID if enabled.
{
"data": [
{
"statements": [
{
"date": "2020-06-15T12:00:00.000Z",
"payPeriodStartDate": "2020-05-27T12:00:00.000Z",
"payPeriodEndDate": "2020-06-12T12:00:00.000Z",
"grossAmount": 1000,
"ytdGrossAmount": 10000,
"netAmount": 800,
"ytdNetAmount": 8000,
"paymentMethod": "deposit",
"hours": 37,
"deductions": [
{
"category": "taxes",
"label": "Federal Income Tax",
"rawLabel": "Federal Income Tax",
"amount": 200,
"ytdAmount": 2000
},
{
"category": "taxes",
"label": "State Income Tax",
"rawLabel": "Utah State Tax",
"amount": 50,
"ytdAmount": 500
},
{
"category": "other",
"label": "Abc corp dd",
"rawLabel": "Abc corp dd",
"amount": 5,
"ytdAmount": 50
}
],
"earnings": [
{
"category": "benefit",
"rawLabel": "Social Security (Disability)",
"amount": 1000
},
{
"category": "bonus",
"rawLabel": "Quarterly Bonus",
"amount": 2000,
"ytdAmount": 6000
},
{
"category": "overtime",
"rawLabel": "Overtime Pay",
"amount": 100,
"ytdAmount": 1000,
"hours": 10,
"rate": 15
},
{
"category": "reimbursement",
"rawLabel": "Gas Card",
"amount": 25.47,
"ytdAmount": 85.74
}
],
"netAmountAdjustments": [
{
"label": "Mileage Reimbursement",
"amount": 25
}
],
"paystub": {
"_id": "60abeff50836730008616fad",
"url": "[ATOMIC-GENERATED-PRESIGNED-S3-URL]"
},
"parsedData": {
"date": "2020-06-15T12:00:00.000Z",
"payPeriodStartDate": "2020-05-27T12:00:00.000Z",
"payPeriodEndDate": "2020-06-12T12:00:00.000Z",
"grossAmount": 1000,
"ytdGrossAmount": 10000,
"netAmount": 800,
"ytdNetAmount": 8000,
"paymentMethod": "deposit",
"hours": 37,
"deductions": [
{
"category": "taxes",
"rawLabel": "Federal Income Tax",
"amount": 200,
"ytdAmount": 2000
},
{
"category": "taxes",
"rawLabel": "Utah State Tax",
"amount": 50,
"ytdAmount": 500
},
{
"category": "other",
"rawLabel": "Abc corp dd",
"amount": 5,
"ytdAmount": 50
}
],
"earnings": [
{
"category": "benefit",
"rawLabel": "Social Security (Disability)",
"amount": 1000
},
{
"category": "bonus",
"rawLabel": "Quarterly Bonus",
"amount": 2000,
"ytdAmount": 6000
},
{
"category": "overtime",
"rawLabel": "Overtime Pay",
"amount": 100,
"ytdAmount": 1000,
"hours": 10,
"rate": 15
},
{
"category": "reimbursement",
"rawLabel": "Gas Card",
"amount": 25.47,
"ytdAmount": 85.74
}
],
"netAmountAdjustments": [
{
"rawLabel": "Mileage Reimbursement",
"amount": 25
}
]
}
},
{
"date": "2020-06-30T12:00:00.000Z",
"payPeriodStartDate": "2020-05-27T12:00:00.000Z",
"payPeriodEndDate": "2020-06-12T12:00:00.000Z",
"grossAmount": 1000,
"paymentMethod": "check",
"hours": 34,
"deductions": [
{
"category": "taxes",
"label": "Federal Income Tax",
"rawLabel": "Federal Income Tax",
"amount": 200
},
{
"category": "taxes",
"label": "State Income Tax",
"rawLabel": "Utah State Tax",
"amount": 50
},
{
"category": "other",
"label": "Abc corp dd",
"rawLabel": "Abc corp dd",
"amount": 5
}
],
"paystub": {
"_id": "60abeff50836730008616fae",
"url": "[ATOMIC-GENERATED-PRESIGNED-S3-URL]"
}
}
],
"company": {
"_id": "5d77f9e1070856f3828945c6",
"name": "DoTerra",
"branding": {
"logo": {
"_id": "5eb62781b4b83f0008f638cc",
"url": "https://cdn.atomicfi.com/doterra-logo.svg"
}
}
},
"connector": {
"_id": "5d77f95207085632a58945c3",
"name": "ADP",
"branding": {
"logo": {
"_id": "5eb62781b4b83f0008f6343c",
"url": "https://cdn.atomicfi.com/adp-logo.svg"
}
}
},
"linkedAccount": "5f7212103a40e91f95ba376d",
"task": "602414d84f9a1980cf5eafcc"
}
]
}
Timesheets
Get timesheets data once its been synced to Atomic from the payroll provider.
For purely Deposit switch use cases, this information is not collected.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
Required Properties
identifier
string- The user's unique identifier, as sent to Atomic during AccessToken creation.
Optional Properties
linkedAccount
string
_id
of a LinkedAccount object provided as a query string parameter.task
string
_id
of the task, provided as a query string parameter, that was used to retrieve the data. If this parameter is not provided, the data that was most recently retrieved from the payroll system will be returned.includeOutputMetadata
string
true
, the outputMetadata
will be included in the response.GET
/timesheets/:identifierResponse
A successful request will return the timesheets for the user.
data
[Timesheet]- An array of objects that include a timesheets object,
company
,connector
,task
ID, andlinkedAccount
ID if enabled.
{
"data": [
{
"timesheets": [
{
"duration": 420,
"date": "2021-10-13T12:00:00.000Z",
"type": "unpaid",
"clockedIn": "2021-10-13T13:00:00.000Z",
"clockedOut": "2021-10-13T20:00:00.000Z"
},
{
"duration": 480,
"date": "2021-10-12T12:00:00.000Z",
"type": "paid",
"clockedIn": "2021-10-12T13:00:00.000Z",
"clockedOut": "2021-10-12T21:00:00.000Z"
},
{
"duration": 340,
"date": "2021-10-11T12:00:00.000Z",
"type": "paid",
"clockedIn": "2021-10-11T13:00:00.000Z",
"clockedOut": "2021-10-11T18:40:00.000Z"
}
],
"company": {
"_id": "5d77f9e1070856f3828945c6",
"name": "DoTerra",
"branding": {
"logo": {
"_id": "5eb62781b4b83f0008f638cc",
"url": "https://cdn.atomicfi.com/doterra-logo.svg"
}
}
},
"connector": {
"_id": "5d77f95207085632a58945c3",
"name": "ADP",
"branding": {
"logo": {
"_id": "5eb62781b4b83f0008f6343c",
"url": "https://cdn.atomicfi.com/adp-logo.svg"
}
}
},
"linkedAccount": "5f7212103a40e91f95ba376d",
"task": "602414d84f9a1980cf5eafcc"
}
]
}
Taxes
Get tax data once its been synced to Atomic from the payroll provider.
For purely Deposit switch use cases, this information is not collected.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
Required Properties
identifier
string- The user's unique identifier, as sent to Atomic during AccessToken creation.
Optional Properties
linkedAccount
string
_id
of a LinkedAccount object provided as a query string parameter.task
string
_id
of the task, provided as a query string parameter, that was used to retrieve the data. If this parameter is not provided, the data that was most recently retrieved from the payroll system will be returned.includeOutputMetadata
string
true
, the outputMetadata
will be included in the response.GET
/taxes/:identifierResponse
A successful request will return the taxes for the user.
data
[Tax]- An array of objects that include a array of taxes objects,
company
,connector
,task
ID, andlinkedAccount
ID if enabled.
{
"data": [
{
"taxes": [
{
"type": "w2",
"year": "2020-01-01T00:00:00.000Z",
"totalWages": 50000,
"form": {
"_id": "60abeff60836730008616faf",
"url": "[ATOMIC-GENERATED-PRESIGNED-S3-URL]"
},
"parsedData": {
"taxYear": 2022,
"employeeTin": "XXX-XX-1234",
"employerTin": "12-3456789",
"employerNameAddress": {
"name1": "Tax Form Issuer, Inc",
"line1": "12021 Sunset Valley Dr",
"line2": "Suite 230",
"city": "Preston",
"state": "VA",
"postalCode": "20191"
},
"controlNumber": "012547 WY/OA7",
"employeeName": {
"first": "Kris",
"last": "Public"
},
"employeeAddress": {
"line1": "1 Main St",
"line2": "Apartment 123",
"city": "Melrose",
"state": "NY",
"postalCode": "12121"
},
"wages": 44416.74,
"federalTaxWithheld": 6907.16,
"socialSecurityWages": 47162.92,
"socialSecurityTaxWithheld": 2924.1,
"medicareWages": 47162.92,
"medicareTaxWithheld": 683.86,
"socialSecurityTips": 134.25,
"allocatedTips": 149.75,
"dependentCareBenefit": 543.25,
"nonQualifiedPlan": 354.23,
"codes": [
{
"code": "C",
"amount": 301.5
},
{
"code": "D",
"amount": 2746.18
},
{
"code": "DD",
"amount": 4781.88
}
],
"other": [
{
"description": "Housing",
"amount": 6500
},
{
"description": "Union Dues",
"amount": 1500
}
],
"statutory": false,
"retirementPlan": true,
"thirdPartySickPay": false,
"stateTaxWithholding": [
{
"stateTaxWithheld": 1726.78,
"state": "OH",
"stateTaxId": "OH 036-133505158F-01",
"stateIncome": 44416.74
}
],
"localTaxWithholding": [
{
"localTaxWithheld": 427.62,
"localityName": "Kirtland",
"state": "OH",
"localIncome": 44416.74
}
]
}
},
{
"type": "1099",
"year": "2020-01-01T00:00:00.000Z",
"form": {
"_id": "60abeff60836730008616faf",
"url": "[ATOMIC-GENERATED-PRESIGNED-S3-URL]"
}
}
],
"company": {
"_id": "5d77f9e1070856f3828945c6",
"name": "DoTerra",
"branding": {
"logo": {
"_id": "5eb62781b4b83f0008f638cc",
"url": "https://cdn.atomicfi.com/doterra-logo.svg"
}
}
},
"connector": {
"_id": "5d77f95207085632a58945c3",
"name": "ADP",
"branding": {
"logo": {
"_id": "5eb62781b4b83f0008f6343c",
"url": "https://cdn.atomicfi.com/adp-logo.svg"
}
}
},
"linkedAccount": "5f7212103a40e91f95ba376d",
"task": "602414d84f9a1980cf5eafcc"
}
]
}
Linked Account
A LinkedAccount
is a persistent connection that has been authenticated by an end-user. Once established, it can be used to perform read and write operations. Linked Accounts are the mechanism we use to facilitate our Continuous Access product. They are also used to initiate Task Workflows in the background.
List Linked Accounts
For a Continuous Access workflow, use this endpoint to list accounts linked to a particular user.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
Required Properties
identifier
string- The user's identifier, as sent to Atomic during AccessToken creation. Passed with the URL as a path parameter (e.g.
/linked-account/list/:identifier
), replacing:identifier
with your user'sidentifier
.
GET
/linked-account/list/:identifierResponse
The response contains an array of Linked Account objects for the end user.
_id
string- Unique identifier for the linked account.
valid
boolean- Whether or not the account credentials were valid after the last attempted use.
transactRequired
boolean- Whether or not using the account requires the user to be present within Transact.
lastSuccess
string- The datetime of the last successful usage of the account in ISO 8601 format.
lastFailure
string- The datetime of the last failed usage of the account in ISO 8601 format.
company
object- The Company to which the account is linked.
connector
object- The Connector to which the account is linked.
{
"data": [
{
"_id": "5f7212103a40e91f95ba376d",
"valid": true,
"connector": {
"_id": "5d77f95207085632a58945c3",
"name": "ADP",
"branding": {}
},
"company": {
"_id": "5d77f9e1070856f3828945c6",
"name": "DoTerra",
"branding": {
"logo": {
"_id": "5eb62781b4b83f0008f638cc",
"url": "https://cdn.atomicfi.com/logo.svg"
}
}
},
"lastSuccess": "2020-09-28T16:40:48.009Z",
"transactRequired": false
}
]
}
Use a Linked Account
Use this endpoint to initiate a Task Workflow in the background with a Linked Account. Send a request to this endpoint containing an array of the tasks
you wish to execute and the _id
of the LinkedAccount
. This is generally used to refresh the data sync'ed to Atomic from a payroll system.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
Required Properties
tasks
[TaskConfiguration]- Defines configuration for the tasks you wish to execute as part of the task workflow.
Child Properties
Required Properties
operation
string- Specifies the operation with which to initialize Transact. One of
deposit
,verify
, ortax
. product
stringDeprecated- One of
deposit
,verify
, ortax
. This is deprecated in favor ofoperation
.product
will be available for the foreseeable future and we will give ample warning when it is planned to be sunset.
Optional Properties
onComplete
stringThe action to take on completion of the task. Can be either "continue" or "finish." To execute the next task, use "continue." To finish the task workflow and not execute any of the subsequent tasks, use "finish."Default value: "continue"
onFail
stringThe action to take on failure of the task. Can be either "continue" or "finish." To execute the next task, use "continue." To finish the task workflow and not execute any of the subsequent tasks, use "finish."Default value: "continue"
distribution
objectOptionally pass in enforced deposit settings.Child Properties
Required Properties
type
string- Can be
total
to indicate the remaining balance of their paycheck,fixed
to indicate a specific dollar amount, orpercent
to indicate a percentage of their paycheck.
Optional Properties
amount
numberWhen
distribution.type
isfixed
, it indicates a dollar amount to be used for the distribution. Whendistribution.type
ispercent
, it indicates a percentage of a paycheck. This is not required ifdistribution.type
istotal
.This value cannot be updated by the user unless
canUpdate
is set totrue
. linkedAccount
string- The
_id
of a LinkedAccount object.
POST
/task-workflow/from-linked-accountResponse
Successfully creating a Task Workflow will return a payload with a data
object containing metadata about the created taskWorkflow
, its first task
, and the associated company
. The Task List in the Atomic Console can be used to track task progress.
Subsequent Tasks after the task
referenced in the response will be processed as needed depending on the results of the first Task as well as the configuration provided; their progress may be tracked via webhooks. If you plan on implementing webhooks, we recommend saving the _id
values of the task
and the taskWorkflow
for reference.
task
object- Metadata for the first task in the task workflow.
taskWorkflow
object- Metadata for the created task workflow.
company
object- Metadata for the end user's company or payroll provider.
Child Properties
{
"data": {
"task": {
"_id": "5d3b23b155f500465c895f60"
},
"taskWorkflow": {
"_id": "5d3b23b155f500465c895f5f"
},
"company": {
"_id": "5d77f9e1070856f3828945c6",
"name": "Mocky",
"branding": {
"logo": {
"url": "https://cdn-public.atomicfi.com/979115f4-34a0-44f5-901e-753a33337444_atomic-logo-dark.png"
},
"color": "#090721"
}
}
}
}
User
A User
is an entity in the Atomic system that is created during the access token creation process. Details about a user's accounts are stored on the user object for reference as needed in an operation; ie. for adding a deposit account to a payroll system or updating the card-on-file for a merchant service.
End Monitoring
This endpoint is used to remove monitoring for users that have payroll data monitoring enabled via continuous access.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
Required Properties
identifier
string- The user's unique identifier, as sent to Atomic during AccessToken creation.
PUT
/user/:identifier/end-monitoring{
"success": true
}
Get Tasks
This endpoint is used to retrieve tasks for the specified user.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
Required Properties
identifier
string- The user's unique identifier, as sent to Atomic during AccessToken creation.
Optional Properties
status
string
queued
, processing
, failed
, or completed
.payrollDataAvailable
string
GET
/user/:identifier/tasksResponse
A successful response will return an object with data.tasks
equal to an array of task objects with the following properties.
_id
string- The id of the task.
authenticated
boolean- A boolean value indication whether or not the task has successfully authenticated against the payroll system.
createdAt
date- The timestamp when the task was created, stored in UTC and ISO 8601 format.
failReason
stringOptional- The failure reason associated with a failed task. Will consist of one of the failure values.
payrollDataAvailable
boolean- A boolean value indicating whether or not the user's payroll data is ready for retrieval via api.
product
enum- The product relevant to the executed task. Options are:
deposit
verify
status
string- The status of the task which may be
queued
,processing
,failed
, orcompleted
.
{
"data": {
"tasks": [
{
"_id": "65b3f3c8b871a9626c36d103",
"authenticated": true,
"createdAt": "2024-01-26T18:02:55.795Z",
"payrollDataAvailable": true,
"product": "verify",
"status": "completed"
},
{
"_id": "65b3f3c8b871a9626c36d103",
"authenticated": true,
"createdAt": "2024-01-26T18:02:55.795Z",
"failReason": "bad-credentials",
"payrollDataAvailable": false,
"product": "deposit",
"status": "failed"
}
]
}
}
Update User
This endpoint is used to add data to a user in response to an onDataRequest
event emitted from our Transact SDK. Any data passed will be upserted into the user's object. The typical use case is to delay the transmission of sensitive data (bank accounts, cards, identity information, etc.) until our system has need for such data.
The shape of the request body is similar to our access token creation endpoint, except the data to be added/updated will be nested in a data
key, and the identifier
will not be nested, but remain on the first-level of the object.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
PUT
/userWebhooks
Webhooks are the recommended way to receive data and updates from the Atomic system. They allow us to send real-time updates as user-driven events occur within the Atomic system to an API endpoint in your system via HTTPS POST calls. All webhooks are secured via an API Secret used to sign the webhook with an HMAC signature which is included in the header of the HTTPS call. Refer to our How to Secure Webhooks guide for more details.
Webhook endpoints can be configured in the Atomic Console or via API.
Create Endpoint
This API is used to register a webhook endpoint for receiving updates from the Atomic system.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
Required Properties
url
string- The URL of the endpoint where Atomic will send webhook events for the associated webhook event types.
secretId
string- The ID of the API secret that will be used to create the HMAC signature of the webhook event.
eventTypes
[string]- A list of webhook event types that the endpoint will listen for.
POST
/webhooks/endpointsResponse
A successful response will return a JSON object with data.endpoint
equal to the newly created endpoint object along with the _id
field for the new webhook endpoint.
{
"data": {
"endpoint": {
"url": "https://your-endpoint.com/webhooks",
"secretId": "65c6adc38a19739b40d68a60",
"eventTypes": [
"task-authentication-status-updated",
"task-status-updated"
],
"_id": "65cd12a0fee167d71e442b1b"
}
}
}
Get Endpoints
This endpoint is used to retrieve the configured webhook endpoints.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
GET
/webhooks/endpointsResponse
A successful response will return a JSON object with data.endpoints
equal to an array of endpoint objects with the following properties.
_id
string- The ID of the endpoint in the Atomic system.
url
string- The URL of the endpoint where Atomic will send webhook events for the associated webhook event types.
secretId
string- The ID of the API secret that will be used to create the HMAC signature of the webhook event.
eventTypes
[string]- A list of webhook event types that the endpoint will listen for.
{
"data": {
"endpoints": [
{
"_id": "65cd0ead25ae54fd510bc441",
"url": "https://your-endpoint.com/webhooks",
"secretId": "65c6adc38a19739b40d68a60",
"eventTypes": [
"task-status-updated"
]
},
{
"_id": "65cd12a0fee167d71e442b1b",
"url": "https://your-second-endpoint.com/webhooks",
"secretId": "65c6adc38a19739b40d68a60",
"eventTypes": [
"task-authentication-status-updated"
]
}
]
}
}
Update Endpoint
This endpoint is used to update a webhook endpoint which has already been registered in the Atomic system.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
Required Properties
url
string- The URL of the endpoint where Atomic will send webhook events for the associated webhook event types.
secretId
string- The ID of the API secret that will be used to create the HMAC signature of the webhook event.
eventTypes
[string]- A list of webhook event types that the endpoint will listen for.
PUT
/webhooks/endpoints/:_idResponse
A successful response will return a JSON object with data.endpoint
equal to the updated endpoint along with the _id
field.
{
"data": {
"endpoint": {
"url": "https://your-endpoint-updated.com",
"secretId": "65c6adc38a19739b40d68a60",
"eventTypes": [
"task-status-updated"
],
"_id": "65cd12a0fee167d71e442b1b"
}
}
}
Delete Endpoint
This endpoint is used to delete a webhook endpoint from the Atomic system.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
DELETE
/webhooks/endpoints/:_idAPI Secrets
API secrets can be configured in the Atomic Console or via API.
Create API Secret
This endpoint is used to create an API secret in the Atomic system.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
POST
/secretsResponse
A successful response will return a JSON object with data.secret
equal to the newly created secret.
_id
string- The id of the secret.
createdAt
string- The date the secret was created in ISO 8601 format.
name
string- The name of the secret.
token
string- The value of the secret. This is used to make requests to the Atomic api. This value is only viewable in the returned data of this endpoint and in the Atomic console.
{
"data": {
"secret": {
"_id": "65cd3c146e611eb3ebdf4c07",
"createdAt": "2024-02-14T22:17:56.312Z",
"name": "my secret",
"token": "03e87171-cadc-43a7-8c31-a9459d94eb10"
}
}
}
Get API Secrets
This endpoint is used to retrieve all registered API secrets along with the webhook endpoints that they are assigned to.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
GET
/secretsResponse
A successful response will return a JSON object with data.secrets
equal to an array of API secret objects with the following properties.
_id
string- The id of the secret.
createdAt
string- The date the secret was created in ISO 8601 format.
name
string- The name of the secret.
endpoints
[endpoints]- The list of webhook endpoints that the secret is assigned to.
{
"data": {
"secrets": [
{
"_id": "65c6adc38a19739b40d68a60",
"name": "default",
"createdAt": "2024-02-09T22:56:06.842Z",
"endpoints": [
{
"_id": "65cd0ead25ae54fd510bc441",
"url": "https://your-endpoint.com",
"eventTypes": [
"task-status-updated"
]
},
{
"_id": "65cd0ead25ae54fd510bc441",
"url": "https://your-other-endpoint.com",
"eventTypes": [
"task-authentication-status-updated"
]
}
]
},
{
"_id": "65cd3b4595374196666d4d37",
"name": "new secret",
"createdAt": "2024-02-09T22:55:46.240Z",
"endpoints": []
}
]
}
}
Delete API Secret
This endpoint is used to delete an API secret from the Atomic system.
Authentication is handled via the API Key and Secret method. This API is intended to be called from your backend service so your API Keys and Secrets are not deployed to your client side application.
DELETE
/secrets/:_idResponse
A successful response will return a JSON object with success
equal to true
.
{
"success": true
}